using System;
using System.Collections.Generic;
using
System.ComponentModel.DataAnnotations;
using System.Linq;
using System.Web;
namespace MVCSAC.Models
{
public class Account
{
[Required]
[DataType(DataType.Text)]
[Display(Name="Usuário")]
public string Usuario { get; set; }
[Required]
[DataType(DataType.Password)]
[Display(Name="Senha")]
public string Senha { get; set; }
_}
}
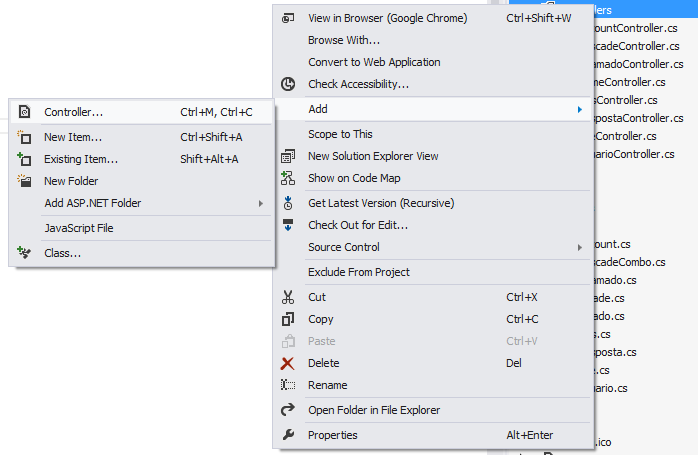
public class AccountController : Controller
{
private iSACContext db =
new iSACContext();
….
}
public_ ActionResult_
LogOut()
_ {
FormsAuthentication.SignOut();
Session.Abandon();
return RedirectToAction("Index", "Home");
private_ List_<string_> consultaPerfil(List_<int_> resposta)
_ {
StringBuilder str = new StringBuilder();
str.Append(@"SELECT PerfilUsu FROM
Usuarios WHERE CHUsu = @CHUsu");
IDataParameter parameter = new SqlParameter();
parameter.DbType = DbType.String;
parameter.ParameterName = "@CHUsu";
parameter.Value = resposta[0];
var perfil = db.Database.SqlQuery<String>(str.ToString(),
parameter).ToList();
return perfil;
}
private List<int> consultaLogOn(Account model)
{
StringBuilder str = new StringBuilder();
str.Append(@"SELECT CHUsu FROM Usuarios
WHERE EMUsu = @EMUsu AND PWUsu = @PWUsu");
IDataParameter parameter = new SqlParameter();
parameter.DbType = DbType.String;
parameter.ParameterName = "@EMUsu";
parameter.Value = model.Usuario;
IDataParameter parameter1 = new SqlParameter();
parameter1.DbType = DbType.String;
_parameter1.ParameterName
= "@PWUsu"_;
_ parameter1.Value = model.Senha;
_
_ var_ resposta = db.Database.SqlQuery<Int32_>(str.ToString(),
parameter, parameter1).ToList();
_ return_ resposta;
}
public_ ActionResult_ LogOn()
_ {
return View();
}
[HttpPost]
public ActionResult LogOn(Account model, string returnUrl)
_{
_ var_ resposta = consultaLogOn(model);
_ if_ (resposta.Count == 0)
_ {
_ ViewBag.Mensagem = "Usuário ou senha inválida, tente novamente!"_;
_ return_ View();
_ }
_ else_
_ {
_ //consulta
perfil_
_ var_ perfil = consultaPerfil(resposta);
_
_ if (perfil != null)
Session.Add("PerfilUsu", perfil[0]);
FormsAuthentication.SetAuthCookie(model.Usuario,
false /* createPersistentCookie */);
Session.Add("Usuario", model.Usuario);
_Session.Add("ChaveUsuario"_,
resposta[0].ToString());
_
_
_ if (Url.IsLocalUrl(returnUrl)
&& returnUrl.Length > 1 && returnUrl.StartsWith("/")
&&
!returnUrl.StartsWith("//") &&
!returnUrl.StartsWith("/\\"))
{
return Redirect(returnUrl);
}
else
{
return RedirectToAction("Index", "Home");
_}
_ }
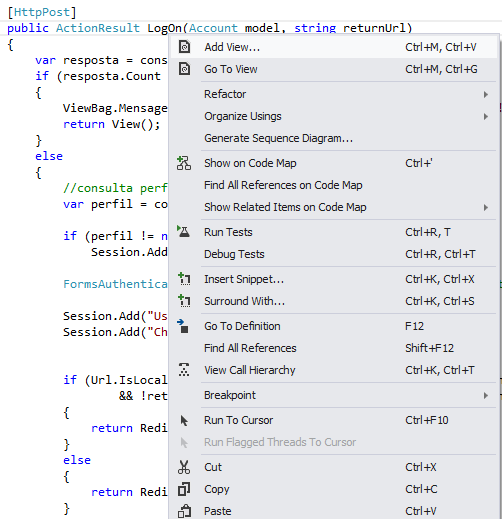
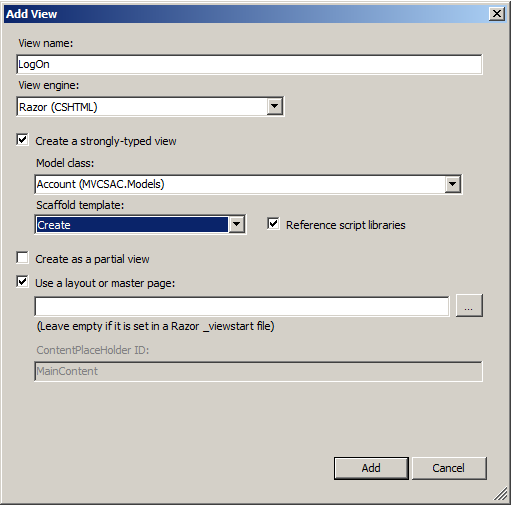
@model MVCSAC.Models.Account
@{
ViewBag.Title = "LogOn";
}
<h2>LogOnh2>
@using (Html.BeginForm()) {
_@_Html.ValidationSummary(true_)
_
_ <fieldset>_
<legend>Accountlegend>
<div class="editor-label">
@Html.LabelFor(model =>
model.Usuario)
div>
<div class="editor-field
focus">
@Html.EditorFor(model =>
model.Usuario)
@Html.ValidationMessageFor(model
=> model.Usuario)
div>
<div class="editor-label">
_@_Html.LabelFor(model => model.Senha)
_ div>
<div class="editor-field">
_@_Html.EditorFor(model => model.Senha)
_ _@_Html.ValidationMessageFor(model => model.Senha)
_ div>
<p>
<input type="submit" value="LogOn" />
<br_ />_
_ <font_ color="red">_@_ViewBag.Mensagemfont>_
_ p>
fieldset>
}
@section Scripts {
@Scripts.Render("~/bundles/jqueryval")
_}_
_
<script_ language="javascript">_
_ //colocando
o foco no botao_
_ document.getElementById("_@_Html.IdFor(model => model.Usuario)"_).focus();
script>_
_
_[Authorize_]
_ public_ class_ RespostaController_ : Controller_
{}
<authentication mode=_"Forms_">_
<forms loginUrl=_"~/Account/LogOn_" timeout=_"2880_" />_
authentication>

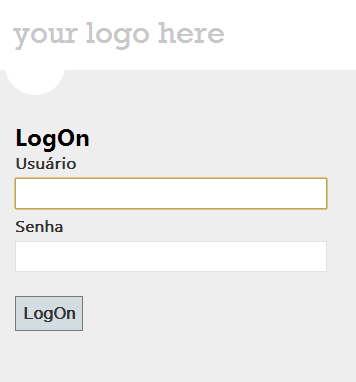
using MVCSAC.DAL;
using MVCSAC.Models;
using System;
using System.Collections.Generic;
using System.Data;
using System.Data.SqlClient;
using System.Linq;
using System.Text;
using System.Web;
using System.Web.Mvc;
using System.Web.Security;
namespace MVCSAC.Controllers
{
public class AccountController : Controller
{
private iSACContext db = new iSACContext();
//
// GET: /Account/
public ActionResult Index()
{
return View();
}
//GET: /Account/LogOut
public ActionResult LogOut()
{
FormsAuthentication.SignOut();
Session.Abandon();
return RedirectToAction("Index", "Home");
}
// GET: /Account/LogOn
public ActionResult LogOn()
{
return View();
}
[HttpPost]
public ActionResult LogOn(Account model, string returnUrl)
_{
_ var_ resposta = consultaLogOn(model);
_ if_ (resposta.Count == 0)
_ {
_ ViewBag.Mensagem = "Usuário ou senha inválida, tente novamente!"_;
_ return_ View();
_ }
_ else_
_ {
_ //consulta
perfil_
_ var_ perfil = consultaPerfil(resposta);
_
_ if (perfil != null)
Session.Add("PerfilUsu", perfil[0]);
FormsAuthentication.SetAuthCookie(model.Usuario,
false /* createPersistentCookie */);
Session.Add("Usuario", model.Usuario);
_Session.Add("ChaveUsuario"_,
resposta[0].ToString());
_
_
_ if (Url.IsLocalUrl(returnUrl)
&& returnUrl.Length > 1 && returnUrl.StartsWith("/")
&&
!returnUrl.StartsWith("//") &&
!returnUrl.StartsWith("/\\"))
{
return Redirect(returnUrl);
}
else
{
return RedirectToAction("Index", "Home");
}
}
}
private List<string> consultaPerfil(List<int> resposta)
{
StringBuilder str = new StringBuilder();
str.Append(@"SELECT PerfilUsu FROM
Usuarios WHERE CHUsu = @CHUsu");
IDataParameter parameter = new SqlParameter();
parameter.DbType = DbType.String;
parameter.ParameterName = "@CHUsu";
parameter.Value = resposta[0];
var perfil =
db.Database.SqlQuery<String>(str.ToString(), parameter).ToList();
return perfil;
}
private List<int> consultaLogOn(Account model)
{
StringBuilder str = new StringBuilder();
str.Append(@"SELECT CHUsu FROM Usuarios
WHERE EMUsu = @EMUsu AND PWUsu = @PWUsu");
IDataParameter parameter = new SqlParameter();
parameter.DbType = DbType.String;
parameter.ParameterName = "@EMUsu";
parameter.Value = model.Usuario;
IDataParameter parameter1 = new SqlParameter();
parameter1.DbType = DbType.String;
_parameter1.ParameterName
= "@PWUsu"_;
_ parameter1.Value = model.Senha;
_
_ var resposta =
db.Database.SqlQuery<Int32>(str.ToString(), parameter, parameter1).ToList();
return resposta;
}
//
// GET: /Account/Create
public ActionResult Create()
{
return View();
}
//
// POST: /Account/Create
[HttpPost]
public ActionResult Create(FormCollection collection)
{
try
{
// TODO: Add insert logic here
return RedirectToAction("Index");
}
catch
{
return View();
_}
_ }
_
_ }
_}